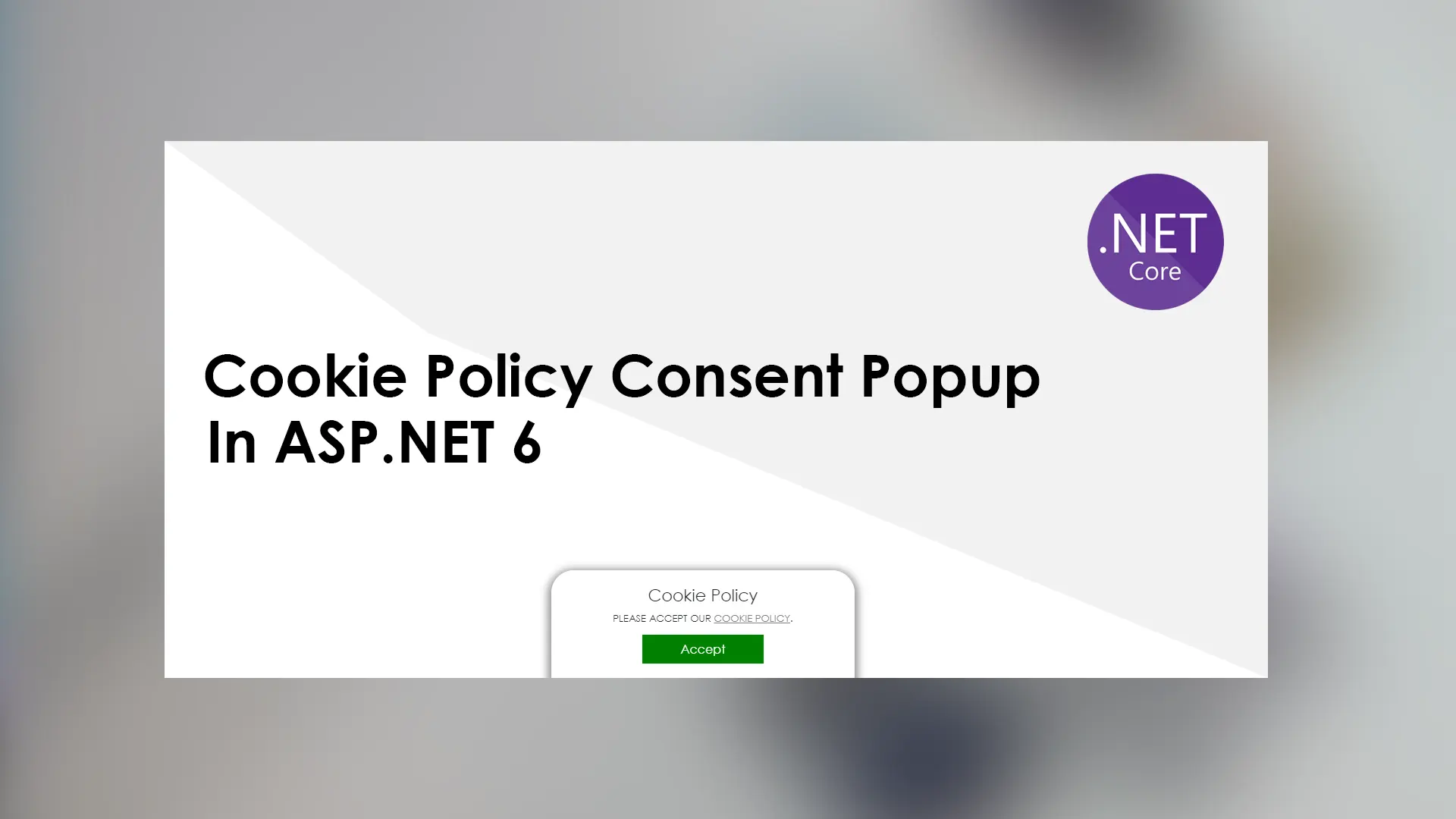
Creating a cookie policy consent popup in ASP.NET 6
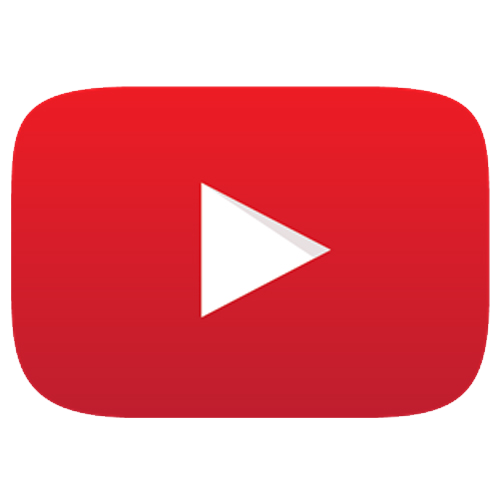
Article have related YouTube video
To comply with the GDPR (General Data Protection Regulation) it is a good idea to implement a cookie consent popup on your website. It is not much code you need in your ASP.NET 6 web app to create a full functional and nicely designed cookie consent popup. In this article I will provide all the code necessary for you. The only thing you need to do yourself is to create the cookie policy page that the popup is linking to.
To keep track of the visitors consent status we will use the ITrackingConsentFeature interface. By using this interface we can easily check the status if the visitor have consent or not by checking for if the cookie of consent exists or not. However first we have to enable ITrackingConsentFeature. In ASP.NET 6 we will need the program.cs file and insert the following code:
Program.cs
builder.Services.Configure<CookiePolicyOptions>(options =>
{
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
......
......
app.UseCookiePolicy();
After enabling the cookie function in our ASP.NET 6 web app, we can then create a partial .cshtml file that will contain the consent popup. So inside the Shared folder, we will create a new file called _CookieConsentPartial.cshtml. The file will look as followed:
_CookieConsentPartial.cshtml
@using Microsoft.AspNetCore.Http.Features
@{
var consentFeatureFlag = Context.Features.Get<ITrackingConsentFeature>();
var showBannerFlag = !consentFeatureFlag?.CanTrack ?? false;
var cookieStr = consentFeatureFlag?.CreateConsentCookie();
}
@if (showBannerFlag)
{
<div id="cookieConsentdiv">
<h3 class="display-6">Cookie Policy</h3>
<p>PLEASE ACCEPT OUR <a asp-page="/CookiePolicy">COOKIE POLICY</a>. </p>
<button type="button" data-cookie-string="@cookieStr">
<span aria-hidden="true">Accept</span>
</button>
</div>
<script type="text/javascript">
$(document).ready(function () {
$("#cookieConsentdiv button[data-cookie-string]").bind("click", function () {
document.cookie = $("#cookieConsentdiv button").attr("data-cookie-string");
$("#cookieConsentdiv").hide();
});
});
</script>
}
In the script there is some jQuery, so we also need to include jQuery library in our project. So go an include this code in your head tag in the _Layout file.
_Layout.cshtml
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
To make the cookie popup box a bit nicer, I made this CSS you are welcome to use:
CSS
#cookieConsentdiv {
z-index: 1000;
position: fixed;
width: 400px;
bottom: 0;
left: 50%;
transform: translateX(-50%);
background-color: rgba(255, 255, 255, 0.9);
padding: 20px 30px 20px 30px;
font-family: 'Century Gothic', sans-serif;
box-shadow: 0px 3px 10px 5px rgba(0, 0, 0, 0.4);
text-align: center;
border-radius: 30px 30px 0 0;
}
#cookieConsentdiv button {
display: block;
margin-left: auto;
margin-right: auto;
border: none;
background-color: green;
padding: 10px 50px;
margin-top: -5px;
color: white;
transition: all ease 0.5s;
}
#cookieConsentdiv button:hover {
background-color: darkgreen;
}
#cookieConsentdiv h3 {
font-size: 22px;
}
#cookieConsentdiv p {
font-size: 13px;
}
#cookieConsentdiv a {
color: gray;
text-decoration: underline;
}
And finally to actually show the popup box we need to include the _CookieConsentPartial.cshtml inside our Shared/_Layout.cshtml file so it gets shown on every page on our website. It doesn't matter where in the _Layout file you put it as long as it is inside the <body> tag. This is because the position of the popup box is set to fixed and have a bottom value of 0. So that will always place it at the bottom of the page.
_Layout.cshtml
<partial name="_CookieConsentPartial" />
Of course you can always customize the popup to match your website design. Enjoy!