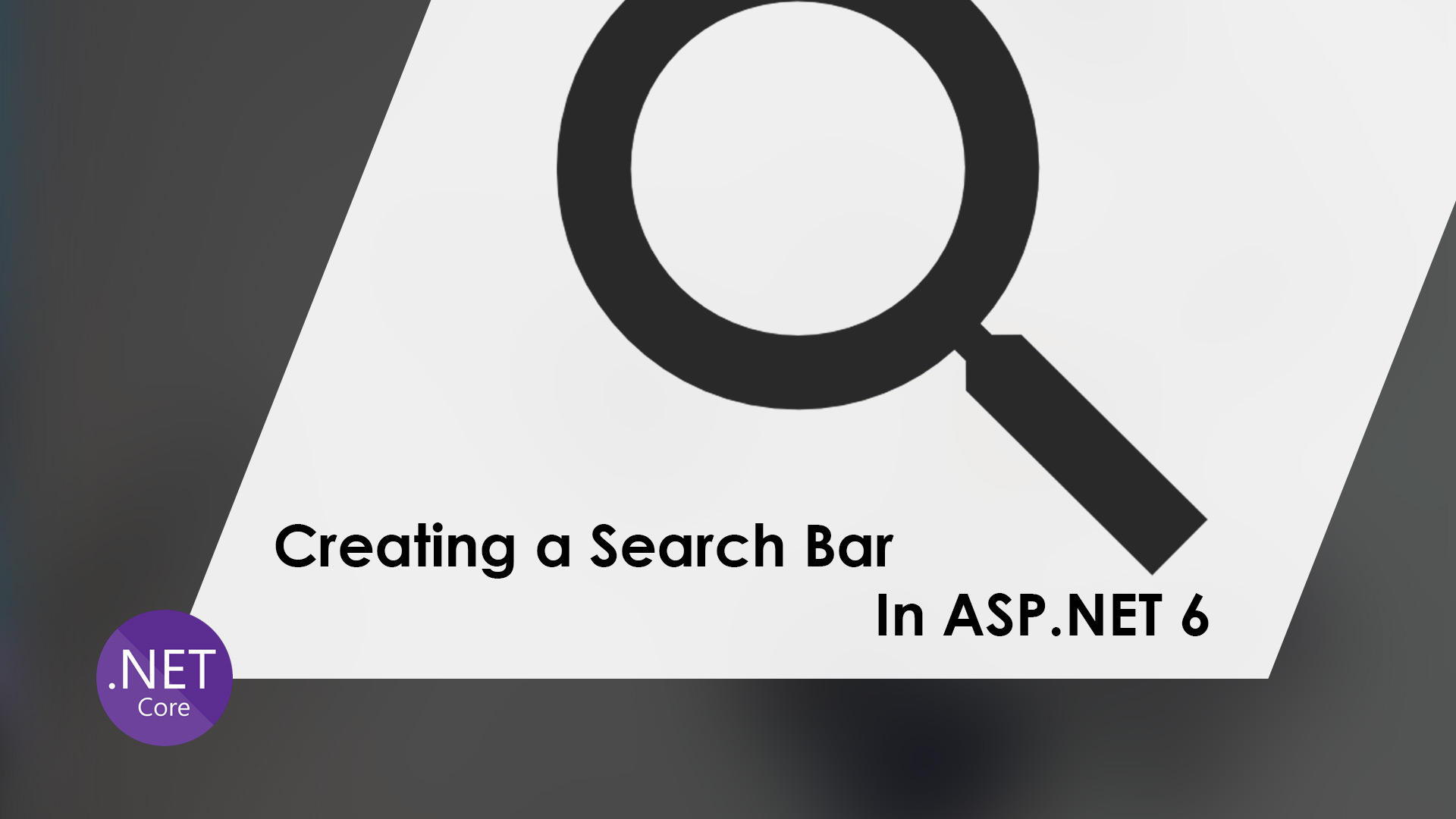
Creating a search bar in ASP.NET 6
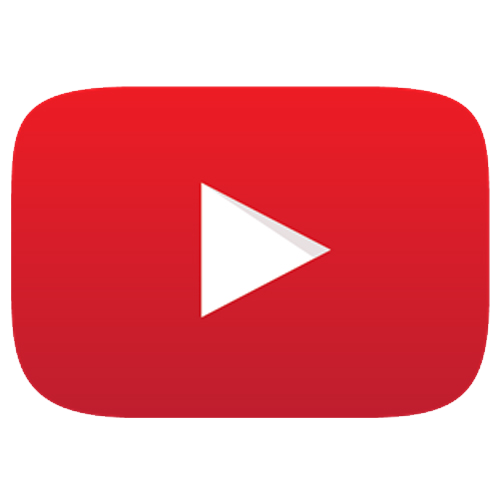
Article have related YouTube video
Creating a search bar on your ASP.NET 6 website is not only just more functionality to your site. It also improves the visitor’s satisfaction-level and the overall SEO of your website. With a search bar people are much more likely to stay on your website no matter if you are running a blog or an e-commerce.
If you want to read more about the benefits of a search bar on your website, go and read this awesome article about search functionality on a website and its SEO benefits.
However, before we begin this article on how to setup a search bar in ASP.NET 6 you already need to have a connection to a database using Entity Framework. Please go and watch my other tutorial on how to setup a connection in MySQL using Entity Framework. You will be able to use whatever SQL technology our want as long it is using Entity Framework.
Let’s begin with the code! First of all, if you haven’t already done it, you need to ensure that you added your context file to the Program.cs file, before being able to fetch data from a database. We do that by adding the dbcontext file to the builders service called AddDbContext<>(). In my case my dbcontext file is called AppDbContext, so the code would look like this:
Program.cs
builder.Services.AddDbContext<AppDbContext>();
In my case I have a model called Article with the properties of Id, Headline and Text. Remember to edit the namespace to your own namespace.
Article.cs
using System.ComponentModel.DataAnnotations;
namespace WebApplication1.Model
{
public class Article
{
[Key]
public int Id { get; set; }
[Required]
public string Headline { get; set; }
[Required]
public string Text { get; set; }
}
}
Next up we want to edit the page where we want the actual search bar to be displayed. The most important thing here is the OnPostAsync method. You can of course also use a GET request instead, however I use a POST request for the search in my example. When there is send a POST request, we want the get the searchString from the request. We are doing that by calling the Request.Form[“searchString”]. It doesn’t need to be called searchString, it highly depends on what name you gave the input in the browser. In my case I call it searchString because it makes sense.
Remember to make a new instance of the List<Article> in the onGet method when the page is loaded. Else you will get a NullReferenceException because the Articles variable is not set to an instance of an object.
In this case I just use the frontpage for the search bar.
Index.cshtml.cs
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using Microsoft.EntityFrameworkCore;
using WebApplication1.Model;
namespace WebApplication1.Pages
{
public class IndexModel : PageModel
{
private AppDbContext _db;
public IndexModel(AppDbContext db)
{
_db = db;
}
public List<Article> Articles { get; set; }
public void OnGet()
{
Articles = new List<Article>();
}
public async Task OnPostAsync()
{
var searchString = Request.Form["searchString"];
Articles = await _db.Article.Where(x => x.Headline.Contains(searchString)).ToListAsync();
}
}
}
Finally, we need to loop through the Articles in the Razor Page file to display the result. This code is not visually optimized however you get a great idea of the functionality behind how the search bar works.
Index.cshtml
@page
@model IndexModel
@{
ViewData["Title"] = "Home page";
}
<div class="text-center">
<h1 class="display-4">Welcome</h1>
<p>Learn about <a href="https://docs.microsoft.com/aspnet/core">building Web apps with ASP.NET Core</a>.</p>
</div>
<form method="post" class="searchForm">
<input type="text" name="searchString" />
<input type="submit" value="Search" />
</form>
@foreach(var article in Model.Articles)
{
<p>@article.Headline</p>
<p>@article.Text</p>
<br />
<br />
<br />
}
That is all the code you need to have a functional search bar. If you have an e-commerce, you can use products instead of articles. Only the creativity sets it limits to what you can search through.