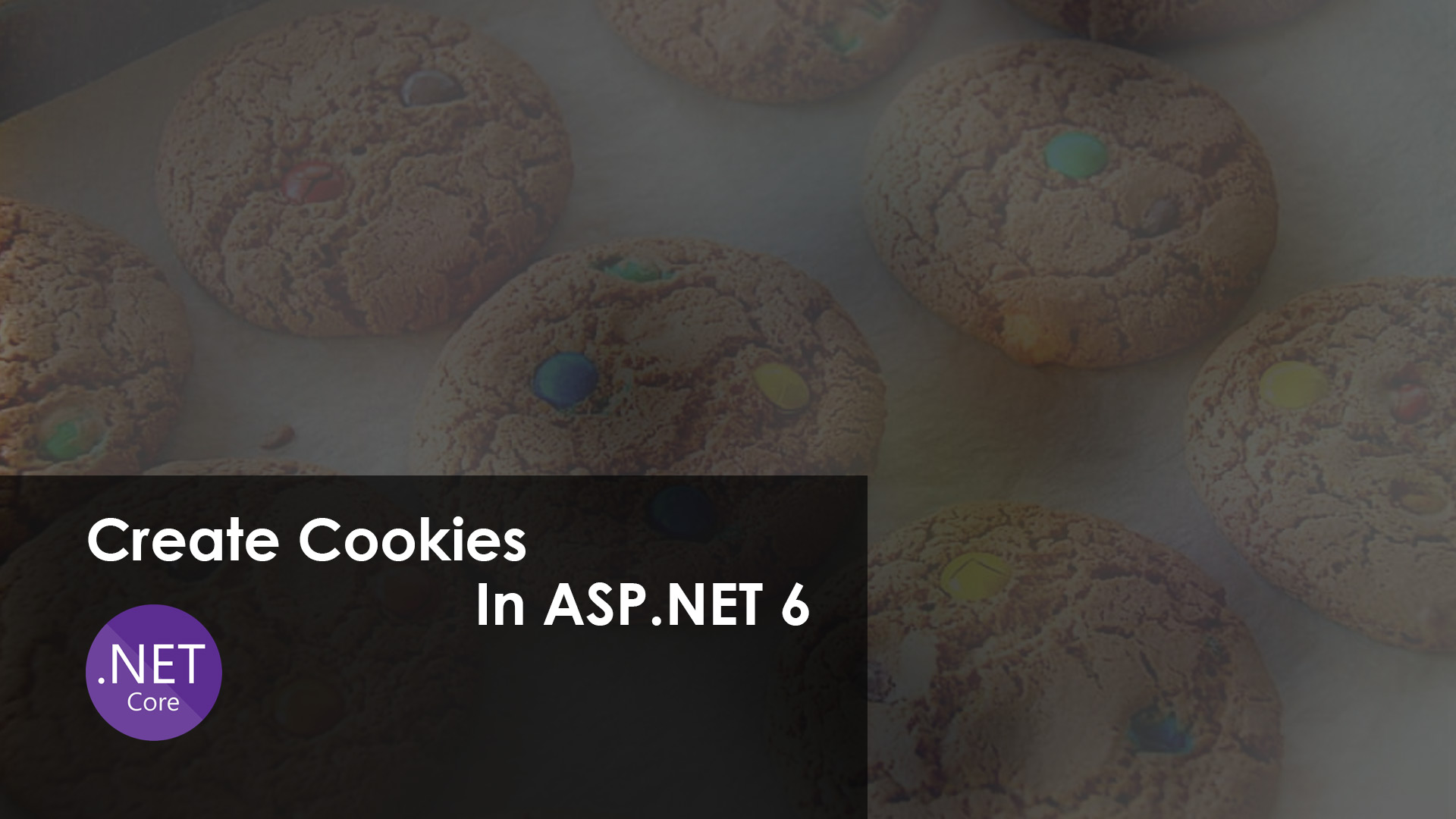
Creating Cookies in ASP.NET 6
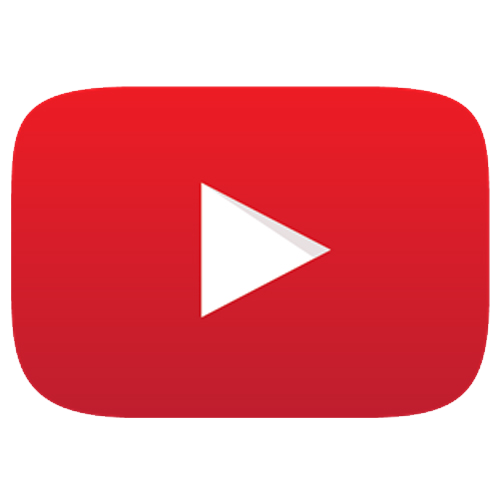
Article have related YouTube video
Cookies is used for multiple purposes on a website and is essential for remembering who the visiting user is. It can be a cookie for a token that will recognize a specific cart for a shopper in a webshop, or a token for some users login session.
There is multiple ways to add cookies to a ASP.NET 6 web app. You could use JS, however in this article I show you how you do it in plain C# using the .NET 6 framework itself.
First I will show you how to do it in a code-behind file like Index.cshtml.cs. After that I will show you how to do it in the _Layout.cshtml file. The _Layout.cshtml example will also work if you are using Blazor.
Index.cshtml.cs
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
namespace WebApplication8.Pages
{
public class IndexModel : PageModel
{
public void OnGet()
{
CookieOptions options = new CookieOptions();
options.Expires = DateTime.Now.AddMonths(1);
options.IsEssential = true;
options.Path = "/";
HttpContext.Response.Cookies.Append("MyCookie", "SDFJKOI20294FI0F0", options);
}
}
}
In a code-behind file the HttpContext already exists because it comes from the extended class PageModel. This means that if you want to do it in the Index.cshtml instead, you can use the exact same code.
However if you want to set a cookie in the _Layout.cshtml file you need to have another approach. Because in the _Layout.cshtml file we do not extend from PageModel. So you need to get the IHttpContextAccessor to get the HttpContext class which will make the cookie.
_Layout.cshtml
@inject IHttpContextAccessor httpContextAccessor
@{
CookieOptions options = new CookieOptions();
options.Expires = DateTime.Now.AddMonths(1);
options.IsEssential = true;
options.Path = "/";
httpContextAccessor.HttpContext.Response.Cookies.Append("MyCookie", "SDFJKOI20294FI0F023432423444444444444444444444", options);
}
To use the IHttpContextAccessor you need to add it as a singleton in the Program.cs file like this under the builder.
Program.cs
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
Deleting Cookie
If you want to delete a cookie, just use the same code as before, but with -1 in DateTime.Now.AddMonths(-1). This will remove the cookie when the code is being executed.