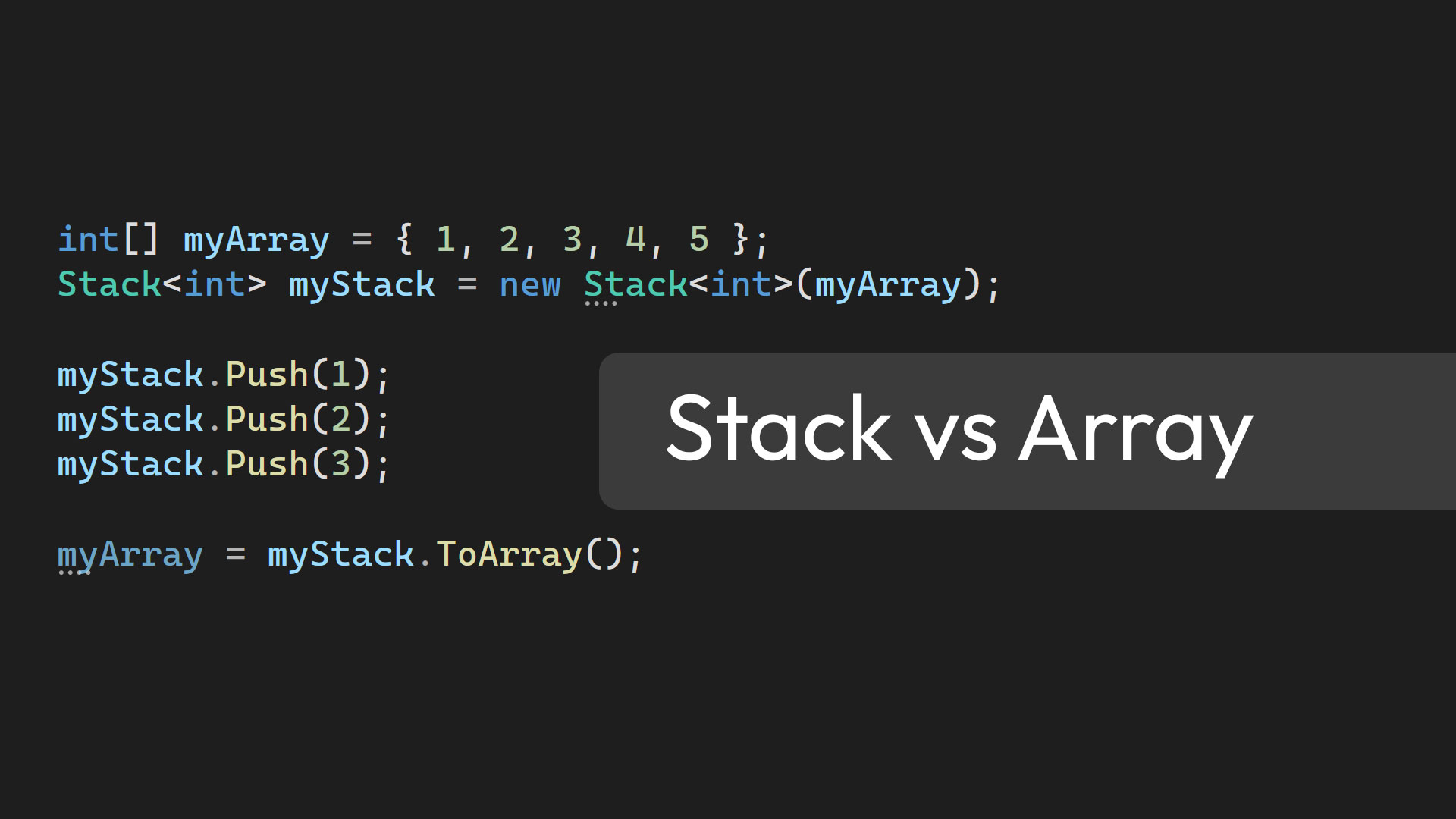
Choosing the right data structure for your project: Stack or Array
When it comes to programming, data structures are the backbone of many applications. One of the most commonly used data structures are stacks and arrays. While they may seem similar at first glance, they have distinct differences and are used for different purposes. In this article, we will take a closer look at these two data structures, exploring their similarities and differences, as well as their use cases. Whether you're a beginner or an experienced developer, this article will provide a comprehensive understanding of stacks and arrays and how to choose the right one for your next project.
When it comes to data structures in programming, it is important to understand the differences between a stack and an array. Both a stack and an array are commonly used in programming, but they are used for different purposes and have some key differences.
An array is a fixed-size collection of elements that are stored in contiguous memory locations. Elements in an array can be accessed by their index, and the size of the array is determined when it is created. Arrays are useful for storing and manipulating large sets of data.
A stack, on the other hand, is a last-in, first-out (LIFO) data structure. This means that the last element added to the stack is the first one to be removed. Stacks are used to implement undo/redo functionality, function calls and return addresses and many more.
Code examples for Stacks and Arrays
Here are some code examples that demonstrate the basic usage of stacks and arrays in C#:
Example of creating and using an array:
Example of creating and using a stack:
The above examples show how to create and use an array and a stack in C#, respectively. The array example demonstrates how to create an array, assign values to its elements, and iterate through the elements. The stack example demonstrates how to create a stack, add elements to it, remove elements from it, and peek at the top element without removing it.
Convert Array to Stack in C#
In the example above, we have an array called myArray containing integers. We create a new stack called myStack and use the constructor that accepts an array as a parameter. This constructor creates a new stack and initializes it with the elements of the array.
Convert Stack to Array in C#
In the example above, we have a stack called myStack containing integers. We use the ToArray() method of the stack to convert it to an array. The ToArray() method returns a new array containing the elements of the stack in the same order as they appear in the stack.
It is important to note that the order of the elements in the array will be the reverse of the order in the stack, as the stack follows LIFO (last in first out) and the array follows the order in which the elements were added.
Also you can use the Array.Reverse() method to reverse the array if you want to have the same order as the stack:
You can also use the List<T> data structure to convert the stack to an array and use the AddRange method to add the elements of the stack to the list, then you can use the ToArray method to convert it to an array.
When it comes to inserting and deleting elements, arrays have a constant time complexity of O(1) when adding or removing elements at the end, while stacks have a time complexity of O(1) for both operations regardless of the position. Stacks are also limited in terms of the number of elements they can hold, whereas arrays can hold a larger number of elements.
In summary, an array is a fixed-size collection of elements that can be accessed by index, whereas a stack is a last-in, first-out data structure that is used for specific use cases like undo/redo and function calls. While arrays and stacks have some similarities, it is important to understand the differences between them so that you can choose the appropriate data structure for your specific programming needs.