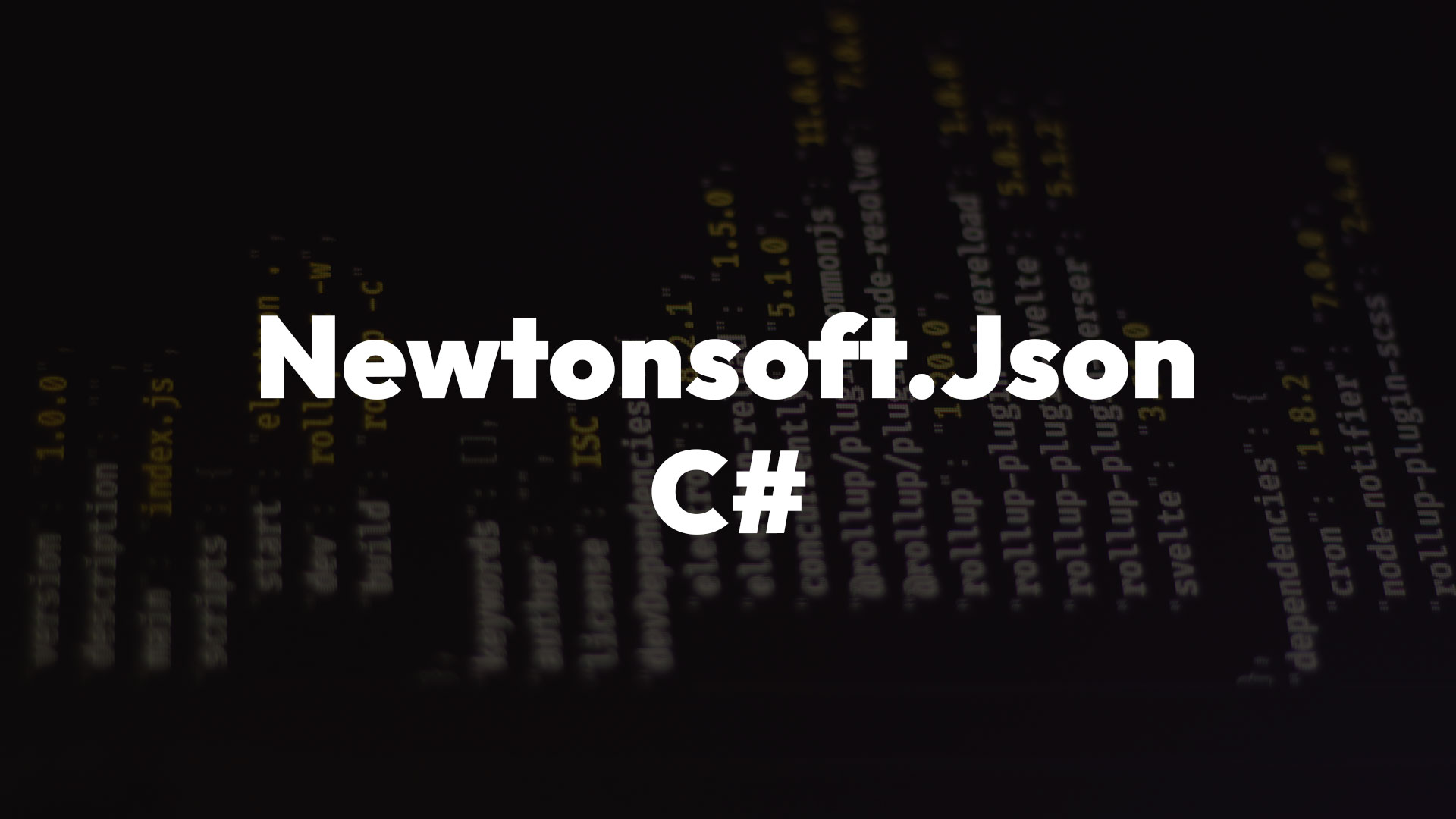
Mastering JSON in C# with Newtonsoft.Json
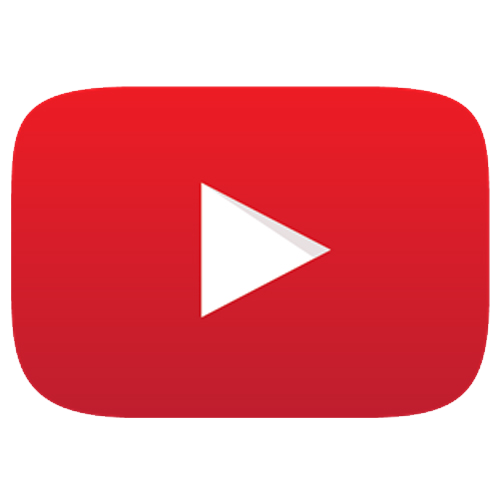
Article have related YouTube video
Dive into the world of Newtonsoft.Json and learn how to work with JSON data in C# like a pro. From installation to advanced features, this guide covers everything you need to know to effectively use this powerful library in your projects and optimize your JSON handling capabilities
Introduction
JSON (JavaScript Object Notation) is a lightweight, human-readable format for storing and exchanging data. It is widely used in web development, mobile development, and other areas where data is exchanged between systems. C# is one of the most popular programming languages for developing applications on the Microsoft platform, and Newtonsoft.Json is a powerful library for working with JSON data in C#.
Newtonsoft.Json, also known as Json.NET, is a popular and widely used library for working with JSON data in C#. It is a high-performance, feature-rich library that makes it easy to parse JSON strings into C# objects, and serialize C# objects back into JSON strings. With support for both .NET Framework and .NET Core, it can be used in a wide range of projects and applications.
The library provides a set of extension methods for working with JSON data and also includes support for LINQ to JSON, which allows you to query and modify JSON data using the LINQ syntax. Additionally, it includes a powerful JsonConverter base class which allows you to easily create custom serialization and deserialization logic. Newtonsoft.Json also supports handling cyclic references, preserving object references and built-in support for handling dates, times, and time zones.
In this article, we will explore the features and capabilities of Newtonsoft.Json and learn how to use it in C# projects. We will start by showing how to install and use the library and then move on to more advanced topics like LINQ to JSON, custom serialization, and performance. By the end of this article, you will have a good understanding of how to use Newtonsoft.Json in your own projects to work with JSON data in C#.
Getting Started
The first step in using Newtonsoft.Json in your C# project is to install the library. The easiest way to do this is by using NuGet, which is a package manager for .NET. To install Newtonsoft.Json using NuGet, open the Package Manager Console in Visual Studio and run the following command:
Once you have installed the library, you can start using it in your code. The main classes you will use are JsonConvert and JObject. JsonConvert contains methods for serializing and deserializing JSON data, and JObject allows you to work with JSON data as a hierarchical object model.
Here's an example of how to parse a JSON string into a C# object using JsonConvert:
And an example of how to serialize a C# object into a JSON string using JsonConvert:
In this example, the Person class is a simple C# class with properties that match the names of the JSON properties in the string. You can also use JObject to work with JSON data as an object model. Here's an example of how to use JObject to access the values of the JSON properties:
This is just a basic example of how to use Newtonsoft.Json to parse and generate JSON in C#. You can also use this library to handle more complex JSON structures and customize the serialization and deserialization process.
You can use this library to read and write JSON files, parse JSON strings and create complex JSON objects. The library is simple to use and has a lot of options that can be set and used to customize the parsing and generating process.
Advanced Features
Newtonsoft.Json offers a wide range of advanced features that allow you to work with JSON data in a more powerful and efficient way. In this section, we will explore some of the key features of the library and learn how to use them in C#.
LINQ to JSON
Newtonsoft.Json provides support for LINQ to JSON, which allows you to query and modify JSON data using the LINQ syntax. This makes it easy to filter, transform, and manipulate JSON data in a way that is similar to how you would work with other LINQ data sources like lists and arrays. Here's an example of how to use LINQ to JSON to filter a JSON array:
JsonConverter
The library includes a powerful JsonConverter base class that allows you to easily create custom serialization and deserialization logic. This can be useful if you need to handle custom types or if you want to control the format of the JSON data. Here's an example of how to create a custom JsonConverter to handle a DateTimeOffset property:
JsonTextReader and JsonTextWriter
These classes allow you to read and write JSON data using a low-level, pull-based API. This can be useful if you need more control over the parsing process or if you want to read JSON data from a non-seekable stream. Here's an example of how to use JsonTextReader to read JSON data from a file:
There are many other advanced features of Newtonsoft.Json, such as JsonSchema, JsonExtensionData, and JsonPath, that allow you to perform even more complex tasks with JSON data. These features provide a wide range of options for working with JSON data in C#, and with the help of Newtonsoft.Json, you can easily handle any JSON-related task you may encounter in your projects.
Custom Serialization
One of the most powerful features of Newtonsoft.Json is its ability to customize the serialization and deserialization process. The library includes a JsonConverter base class that allows you to create custom serialization and deserialization logic for specific types or properties. This can be useful if you need to handle custom types or if you want to control the format of the JSON data.
Here's an example of how to create a custom JsonConverter to handle a custom Point class:
You can then use the custom JsonConverter when serializing or deserializing the Point class:
In addition to custom JsonConverter, you can also use the `JsonObjectAttribute`, `JsonPropertyAttribute`, and `JsonIgnoreAttribute` to customize the serialization and deserialization process on a class or property level. These attributes allow you to specify the name of the JSON property, whether a property should be included in the serialization or deserialization process, and other settings.
In addition to custom JsonConverter, you can also use the `JsonObjectAttribute`, `JsonPropertyAttribute`, and `JsonIgnoreAttribute` to customize the serialization and deserialization process on a class or property level. These attributes allow you to specify the name of the JSON property, whether a property should be included in the serialization or deserialization process, and other settings.
Overall, Newtonsoft.Json's support for custom serialization and deserialization provides a great deal of flexibility and control over how JSON data is handled in C#. With the help of custom JsonConverter and various attributes, you can easily handle any JSON-related task you may encounter in your projects.
Performance
One of the most important factors to consider when working with JSON data is performance. Newtonsoft.Json is a high-performance library that is optimized for speed and memory efficiency. The library uses a lazy parsing approach, which means that it only parses the JSON data as it is needed. This can help reduce memory usage and improve performance when working with large JSON files.
In addition to its lazy parsing approach, Newtonsoft.Json also includes several performance-related features such as:
- The JsonSerializerSettings class allows you to configure various settings such as the maximum depth of the JSON object, the date format, and whether to use the extensible JSON format.
- The JsonSerializer class provides several methods that allow you to perform serialization and deserialization operations without creating an instance of the class each time.
- The JsonTextReader and JsonTextWriter classes allow you to perform low-level, pull-based parsing and writing of JSON data.
In order to optimize performance, it is important to choose the right method for your specific use case. For example, if you need to deserialize a large JSON file, the JsonSerializer.Deserialize method will be faster than the JsonConvert.DeserializeObject method.
It's also important to note that the performance of the library can also be affected by the complexity and size of the JSON data being used. When working with large or complex JSON data, it may be necessary to use a combination of techniques such as lazy loading, caching, and multithreading to achieve optimal performance.
Overall, Newtonsoft.Json is a highly-optimized library that is designed to handle large and complex JSON data with high performance. With its support for lazy parsing, various performance-related features, and the ability to fine-tune the serialization and deserialization process, Newtonsoft.Json provides a powerful and efficient tool for working with JSON data in C#.
Conclusion
Newtonsoft.Json is a powerful and versatile library for working with JSON data in C#. The library provides a wide range of features that allow you to easily handle any JSON-related task you may encounter in your projects. Whether you need to serialize and deserialize JSON data, work with custom types, or customize the serialization and deserialization process, Newtonsoft.Json has you covered.
The library is also designed for high performance, with features such as lazy parsing and various performance-related settings that help to optimize the performance of the library.
In addition, the library is well-documented, actively maintained and widely used in the industry.
Overall, Newtonsoft.Json is a valuable tool for any C# developer working with JSON data, providing a wide range of features, high performance, and ease of use. Whether you're building a new application or adding JSON support to an existing one, Newtonsoft.Json is a solid choice that will help you get the job done quickly and efficiently.