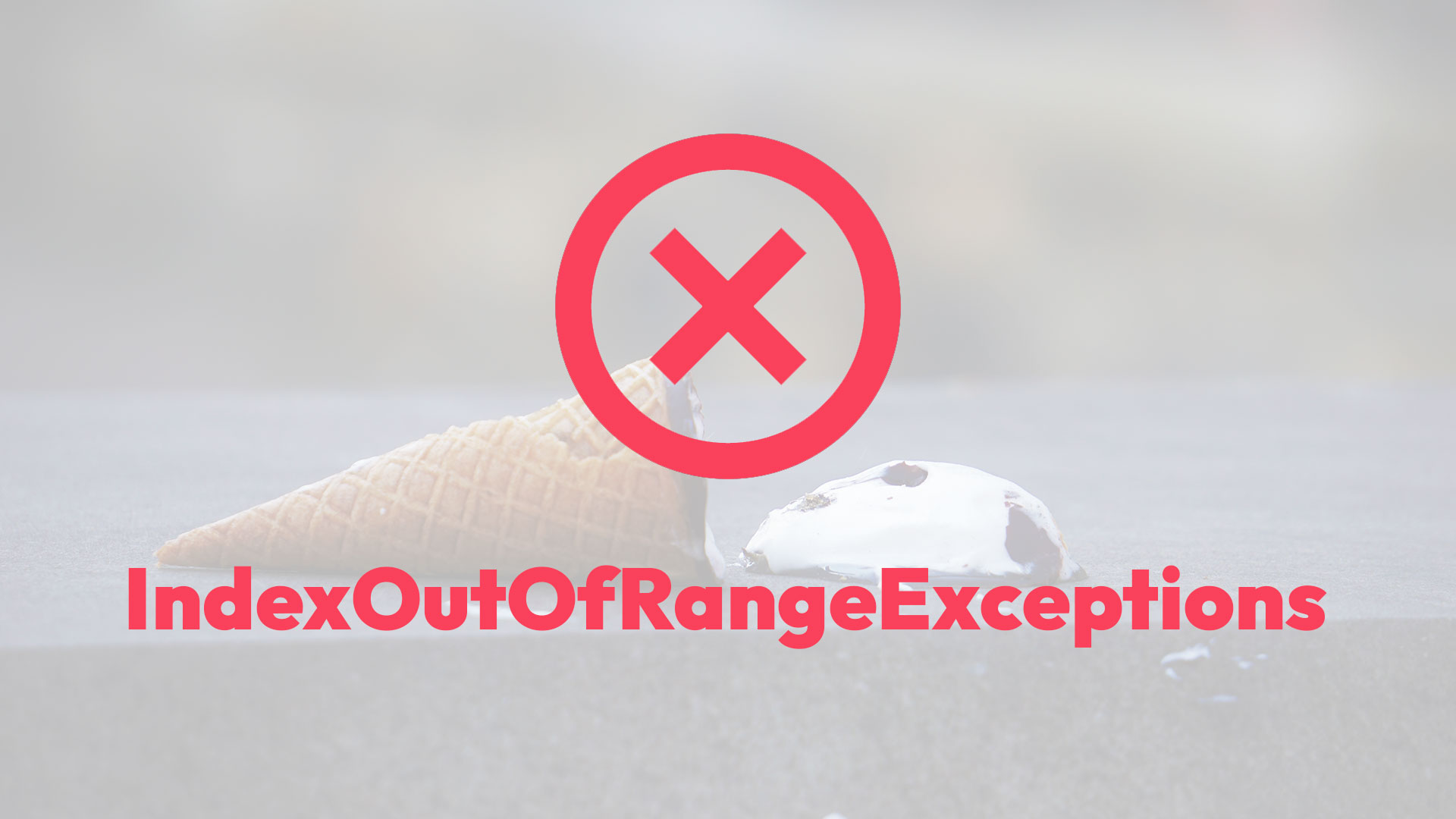
Solving IndexOutOfRangeExceptions in C#
IndexOutOfRangeException is a common error in C# that occurs when trying to access an index that is out of bounds for an array or a list. It can be caused by simple mistakes such as off-by-one errors or uninitialized variables, to more complex issues like unvalidated user input.
An IndexOutOfRangeException is a type of exception that occurs in C# when a program attempts to access an array or list index that is outside of its bounds. This exception is thrown when the index value passed to an array or list is less than zero or greater than or equal to the size of the collection.
There are several common causes of IndexOutOfRangeExceptions in C#. One of the most common causes is when a programmer tries to access an array element using an index that is out of bounds. This can happen when the array is not initialized properly, or when the programmer tries to access an element that does not exist in the array.
Another common cause of IndexOutOfRangeExceptions is when a programmer tries to access a list element using an index that is out of bounds. This can happen when the list is not initialized properly, or when the programmer tries to access an element that does not exist in the list.
A third common cause of IndexOutOfRangeExceptions is when a programmer uses a for loop to iterate over an array or list, and the loop index variable is not properly initialized or incremented. This can cause the loop to run past the end of the array or list, resulting in an IndexOutOfRangeException.
It's also possible that an IndexOutOfRangeException is caused by a bug in the program or by external factors, such as incorrect input provided by the user. It's crucial to keep in mind that an IndexOutOfRangeException is a symptom of a problem, not the problem itself and it's important to identify the underlying cause and fix it.
Troubleshooting and fixing IndexOutOfRangeExceptions in your code
When an IndexOutOfRangeException occurs, it can be challenging to figure out the root cause of the problem. However, there are several steps you can take to troubleshoot and fix the issue.
- Check the exception message: The exception message will typically include the name of the array or list that caused the exception and the index that was accessed. This information can help you to identify the line of code that is causing the problem.
- Check the array or list size: Make sure the array or list is of the correct size and that the index you are trying to access is within the bounds of the collection.
- Check for off-by-one errors: One common mistake that can cause an IndexOutOfRangeException is when a programmer uses an index that is off-by-one. For example, if an array has a length of 10, the valid indices are 0 through 9.
- Check for uninitialized variables: Make sure that any variables used as indices are properly initialized and have valid values.
- Check for incorrect input: If the exception is caused by user input, make sure that the input is validated and that the program can handle input that is out of bounds.
- Check for other exception: Check if there's another exception is being thrown before IndexOutOfRangeException, it could be the root cause of the problem.
Once you have identified the root cause of the problem, you can then take steps to fix it. This may involve modifying your code, changing the way you handle user input, or making other changes to your program. It's important to thoroughly test your code after making changes to ensure that the problem is fully resolved.
In addition to fixing the problem, it's also a good practice to add appropriate error handling and validation code to your program to prevent similar errors from occurring in the future.
Code Examples of IndexOutOfRangeExceptions and their resolutions
Example 1: Array size issue
Resolution:
Example 2: Off-by-one error
Resolution:
Example 3: Uninitialized variable
Resolution:
Example 4: Incorrect input
Resolution:
Conclusion
In conclusion, the IndexOutOfRangeException is a common error that can occur in C# when trying to access an index that is out of bounds for an array or a list. The causes of this error can range from simple mistakes such as off-by-one errors or uninitialized variables, to more complex issues like unvalidated user input.
However, understanding the root cause of the error and using proper troubleshooting techniques can help developers quickly identify and fix the issue. By reviewing the real-world examples and code snippets provided in this article, developers can gain a better understanding of how to handle and prevent IndexOutOfRangeExceptions in their own code.
To avoid this exception it's important to always validate the input and to be aware of the array size and index range. Additionally, it's important to always test and debug the code to ensure that it runs correctly and that any potential issues are identified and corrected before deployment.
In summary, the IndexOutOfRangeException is a common error in C# that can be easily prevented by paying attention to array size, input validation, and debugging. By following these best practices, developers can help ensure that their code runs smoothly and without errors.