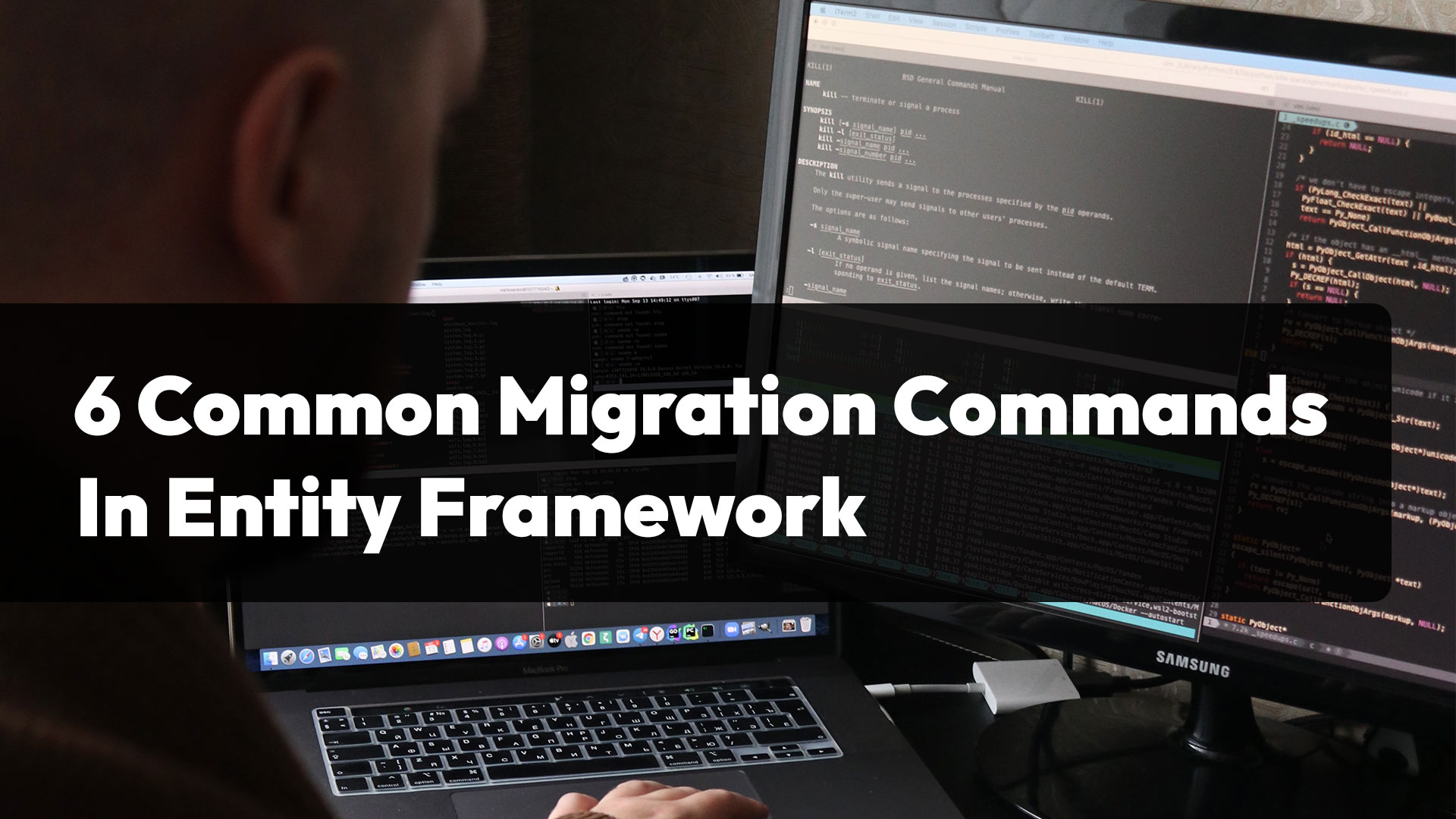
6 common used migration commands in Entity Framework
In this article I will show you the 6 most common commands when migrating with Entity Framework in ASP.NET Core. They will make your life much easier when dealing with SQL and manipulating tables.
These are the 6 migrations i will show you and explain how works:
- Add-Migration
- Update-Database
- Update-Database -TargetMigration:<MigrationName>
- Remove-Migration
- Get-Migrations
- Script-Migration
Add-Migration
The Add-Migration command is a crucial part of Entity Framework migrations. It allows you to create a new migration that captures changes you've made to your model (i.e., entities, relationships, etc.). This command generates code that represents the changes, which you can then use to update your database schema to match the new model.
The Add-Migration command takes a single argument, the name of the migration, which should be a descriptive name that reflects the changes being made. For example, if you're adding a new entity, the name might be "AddProductEntity".
Replace <MigrationName> with a descriptive name for the migration, such as "AddProductEntity" or "UpdateAddressFormat". For example:
This will create a new migration with the specified name, containing code that represents the changes made to your model. The code will be generated in a Migrations folder within your project, and will consist of two files: a .cs file for the migration and a .Designer.cs file for the configuration.
You can then use the Update-Database command to apply the changes to your database schema.
Update-Database
The Update-Database command is an important part of Entity Framework migrations. It's used to apply changes to the database schema, updating it to match the current state of the Entity Framework model.
The Update-Database command updates the database to the latest migration, meaning it will apply all pending migrations in the order they were added. If the database does not exist, Entity Framework will create it. If the database already exists, Entity Framework will modify it to match the latest model.
By default, Entity Framework will try to execute the database changes in a single transaction, so either all changes are applied or none are.
Here is an example of how to use the Update-Database command in the Package Manager Console in Visual Studio:
This will update the database to the latest migration, applying any pending changes to the database schema.
If you want to generate a SQL script instead of executing the changes directly against the database, you can use the -Script option:
This will generate a SQL script that represents the changes to be made to the database, which you can review and execute manually if needed.
You can also update the database to a specific migration by specifying the -TargetMigration option.
Update-Database -TargetMigration:<MigrationName>
Replace <MigrationName> with the name of the migration to which you want to update the database. For example:
This will update the database to the state it was in after the specified migration was applied, rolling back any subsequent migrations if necessary.
Remove-Database
The Remove-Migration command is used in Entity Framework migrations to remove the most recent migration. This is useful if you've made a mistake or changed your mind about a migration you've added and want to start over.
When you remove a migration, Entity Framework will remove the code files for the migration and also update the migration history in the database.
It's important to note that using the Remove-Migration command will not undo any changes that have already been applied to the database. If you've already run the Update-Database command for the migration you want to remove, you'll need to manually revert the changes to the database before removing the migration.
In short, the Remove-Migration command is used to undo the most recent migration in Entity Framework, but it does not undo changes that have already been applied to the database.
Here is an example of how to use the Remove-Migration command in the Package Manager Console in Visual Studio:
This will remove the most recent migration and update the migration history in the database.
Get-Migrations
The Get-Migrations command is used in Entity Framework migrations to view a list of all migrations that have been added to the project.
Here is an example of how to use the Get-Migrations command in the Package Manager Console in Visual Studio:
This will return a list of all migrations in the order they were added, showing the name and timestamp of each migration.
The output of the Get-Migrations command can be helpful in determining which migrations have been applied to the database and which are still pending. You can then use the Update-Database command to apply pending migrations to the database.
Script-Migration
The Script-Migration command is used in Entity Framework migrations to generate a SQL script that represents the changes to be made to the database.
This command can be useful if you want to review or modify the changes to the database schema before applying them. It generates a script that you can execute directly against the database or save for later.
Here is an example of how to use the Script-Migration command in the Package Manager Console in Visual Studio:
This will generate a SQL script that represents the changes to be made to the database by the latest migration.
You can also specify a specific migration to script using the -From and -To options:
Replace <SourceMigration> with the name of the source migration and <TargetMigration> with the name of the target migration. For example:
This will generate a SQL script that represents the changes to be made to the database between the source and target migrations.