C# Basics/
Operators
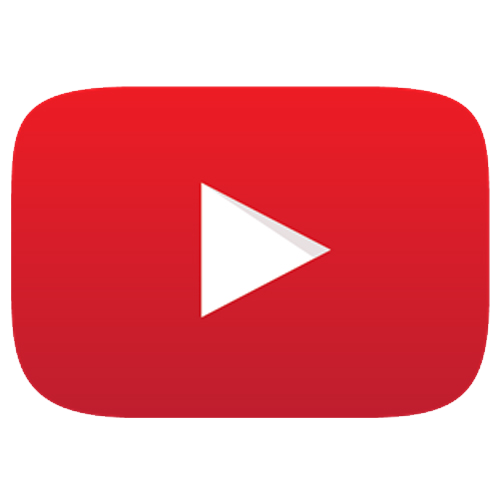
This page have a related YouTube video
- Operators are special symbols that perform specific operations on one or more operands (values or variables).
- C# has a wide range of operators, including arithmetic, assignment, comparison, and logical operators.
- The ternary operator (?:) is a shorthand way to write a simple if-else statement.
- C# also has several other operators, including sizeof, typeof, &, *, and ->, which are used less frequently.
In C#, operators are special symbols that perform specific operations on one or more operands (values or variables). C# has a wide range of operators, including arithmetic, assignment, comparison, and logical operators.
Arithmetic Operators
C# has the following arithmetic operators:
- + (addition)
- - (subtraction)
- * (multiplication)
- / (division)
- % (modulus, or remainder)
For example:
Assignment Operators
C# has the following assignment operators:
- = (assigns a value to a variable)
- += (adds a value to a variable and assigns the result to the variable)
- -= (subtracts a value from a variable and assigns the result to the variable)
- *= (multiplies a variable by a value and assigns the result to the variable)
- /= (divides a variable by a value and assigns the result to the variable)
- %= (calculates the remainder of a variable divided by a value and assigns the result to the variable)
For example:
Comparison Operators
C# has the following comparison operators:
- == (equal to)
- != (not equal to)
- > (greater than)
- < (less than)
- >= (greater than or equal to)
- <= (less than or equal to)
Comparison operators return a boolean value of true or false. For example:
Logical Operators
C# has the following logical operators:
- && (and)
- || (or)
- ! (not)
Logical operators perform boolean logic and return a boolean value. For example:
Conditional Operators
C# has the following conditional operator:
- ?: (ternary operator)
The ternary operator is a shorthand way to write a simple if-else statement. It has the following syntax:
If the condition is true, the trueValue is returned; otherwise, the falseValue is returned. For example:
Other Operators
C# also has several other operators, including the following:
- sizeof (returns the size of a data type in bytes)
- typeof (returns the type of an object or data type)
- & (address-of operator)
- * (dereference operator)
- -> (member access operator for pointers)
These operators are used less frequently and are beyond the scope of this introduction.